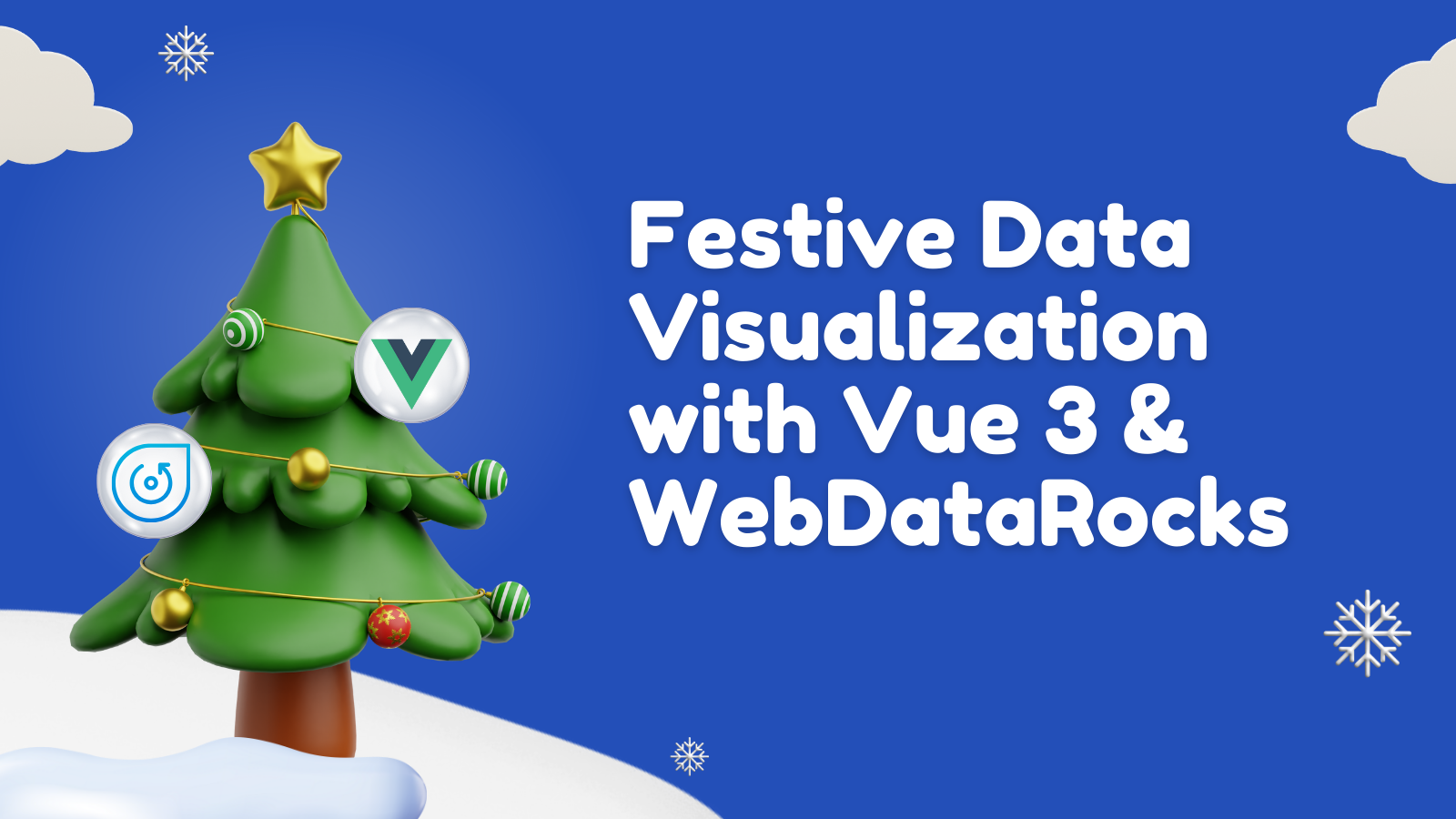
Do You See What We See? A Festive Data Visualization with Vue 3 and WebDataRocks
In this article
With the holidays fast approaching, our team has been brainstorming ideas for meaningful gifts for our colleagues. This year, our workplace is hosting a “Secret Santa” gift exchange, and finding the perfect presents has proven to be quite the challenge. While exploring Kaggle, we came across an intriguing dataset called Customer Shopping (Latest Trends) and […]
With the holidays fast approaching, our team has been brainstorming ideas for meaningful gifts for our colleagues. This year, our workplace is hosting a “Secret Santa” gift exchange, and finding the perfect presents has proven to be quite the challenge. While exploring Kaggle, we came across an intriguing dataset called Customer Shopping (Latest Trends) and thought—what if the data could help?
That’s when we turned to Vue 3 and WebDataRocks—free tools that make it easy to build interactive dashboards and uncover hidden insights. With this JavaScript framework and pivot table library, we’ll explore how various factors, such as gender and season, influence shopping habits. Let’s visualize and analyze the data together—and who knows, maybe we will even find some gift inspiration along the way 🎄
However, before diving into coding, let’s take a look at the result we are aiming for:
Data visualization with Vue 3 and WebDataRocks + Christmas tree animation made with HTML, CSS, and JavaScript
To add a touch of holiday magic, we created an animated Christmas tree to bring festive cheer to the project. Crafting the animation involved a fair amount of CSS, as well as some JavaScript to make the tree interactive. The result is a fun and engaging feature that reacts to user interaction. We’ll dive into a code review later, but for now, we hope it adds a bit of holiday spirit to your day!🎄
Now, get ready to begin the development process. Remember that you can always refer to the source code in the GitHub repository.
But beforehand, check out a quick explanation why Vue + WebDataRocks is a great technology stack.
Why To Choose Vue 3 as a Framework?
Vue 3 is a robust, lightweight framework with a component-based architecture that makes managing and reusing code easy. Its impressive speed comes from the Virtual DOM and optimized reactivity system, which ensures only the necessary updates are made, resulting in faster performance. Additionally, Vue 3 keeps your application’s bundle size small, making it one of the most efficient front-end frameworks available.
Moreover, Vue 3 has well-structured documentation that you can easily refer to whenever needed, making the learning process smoother.
Why To Choose WebDataRocks for Data Visualization?
WebDataRocks is a feature-rich JavaScript pivot table library that perfectly balances functionality, performance, and ease of use. Moreover, WebDataRocks can seamlessly embed into Vue, thus it fits the bill perfectly. In general, this pivot table component supports integration with various frameworks, making it an ideal choice for creating dynamic and interactive data visualization for different applications.
One of the best aspects of this tool is that, despite being free, it offers a robust set of features for data analysis and is highly customizable. While WebDataRocks does have a data size limitation of 1 MB, it remains a powerful and dependable solution for smaller projects and lightweight datasets.
Set Up Vue and WebDataRocks Project
First, to work with any JavaScript framework, you need to have Node.js installed along with your preferred package manager. In this case, we will be using npm.
To create a Vue project and get and include WebDataRocks in it, follow this guide from the official WebDataRocks documentation.
Note that you will be prompted to choose configurations for your project during the creation process. Check the official Vue documentation for more explanation. For simplicity, select No for all configurations.
To ensure the setup works correctly, run this command:
npm run dev
It starts a development server. Open the provided URL in your browser to view the app.
With the project set up, we are ready to dive into coding!
Visualizing Dataset with Vue and WebDataRocks
To get started, let’s import some styles to enhance the pivot table’s appearance.
WebDataRocks comes with various predefined themes, and our team has agreed on the teal one for a clean and vibrant look.
Thus, below you can see how the <template>
section of our App.vue currently looks:
<template>
<header>
<link rel="stylesheet" type="text/css" href="theme/teal/webdatarocks.min.css" />
<div class="main">
<div class="wrapper">
<div class="pivot-container">
<div class="logo">📊 Vue 3 + WebDataRocks</div>
<Pivot toolbar /> //toolbar property set to ‘true’
</div>
</div>
</div>
</header>
</template>
Next, we will define a Report Object as the foundation for building our pivot table. Place it under the <script>
tag in your App.vue file.
However, before configuring the Report Object, download the Customer Shopping (Latest Trends) dataset from Kaggle and save it in your project’s src/assets
folder. Then, link your CSV file to the dataSource
parameter to make the data available for the pivot table.
const reportCsv = {
dataSource: {
filename: 'src/assets/shopping_trends.csv',
},
};
To customize how the data is displayed, configure the Slice Object, which allows you to define rows, columns, and measures, giving you full control over the pivot table structure. For more details on its parameters, refer to the official WebDataRocks documentation.
Thus, our Report Object looks the following way:
const reportCsv = {
dataSource: {
filename: 'src/assets/shopping_trends.csv',
},
slice: {
rows: [
{ uniqueName: 'Category' },
{ uniqueName: 'Item Purchased' },
{ uniqueName: 'Gender' },
],
columns: [
{ uniqueName: 'Season' },
],
measures: [
{
uniqueName: 'Purchase Amount (USD)',
aggregation: 'sum',
},
],
},
options: {
grid: {
showTotals: 'off',
layout: 'classic',
},
},
};
The next step is to pass it to the Pivot component using the report property like this:
<Pivot v-bind:report="reportCsv" toolbar />
And that’s it for setting up the WebDataRocks Pivot Table!
To make the website look more visually appealing, we added the following styles under the <styles scoped>
section in App.vue:
.pivot-container {
min-width: 1000px;
display: flex;
flex-direction: column;
}
For now, the result looks like this:
WebDataRocks Pivot Table component
With the pivot table ready, let’s move on to the next exciting part—creating the Christmas Tree and animating it! 🎄✨
How To Create the Animated Christmas Tree with HTML, CSS and JavaScript
Let’s create a new component named ChristmasTree.vue
, where we’ll set up the tree structure, styles, and behavior. Then in the <template>
section define the HTML structure:
<template>
<!--
- The :class=”{ clicked: isClicked }” dynamically applies a CSS class when clicking the tree.
- The @click and @mouseleave directives bind methods to handle interactions.
-->
<div
id="christmas-tree"
class="christmas-tree"
:class="{ clicked: isClicked }"
@click="toggleTreeState"
@mouseleave="resetTreeState"
>
<div class="tree">
<div class="tree-details"></div>
</div>
<div class="lights">
<div class="row-one"></div>
<div class="row-two"></div>
</div>
<div class="balls"></div>
<div class="star"></div>
<div class="shadow"></div>
</div>
</template>
It’s time to apply CSS to style and animate the Christmas tree. While setting up HTML structure, we have used descriptive names for the section’s classes and ids to keep the code organized and understandable. Since there are many CSS styles involved and space in the blog is limited, you can view the complete code in the ChristmasTree.vue file on my GitHub Repository. Please, don’t just copy the styles but read through them. You know, if there’s an animation property, it’s there for a reason! 😊
We hope you completed the previous step successfully! Now, it’s time to add some interactivity to our Christmas tree using Vue.js. We will start by defining a data property to manage the state. Then, we can include methods to handle toggling and resetting the tree’s state. Last but not least, we’ll use event listeners to create dynamic behavior when the tree is clicked or the mouse leaves the tree area.
Thus, the code should look the following way:
<script>
export default {
data() {
return {
isClicked: false,
};
},
methods: {
toggleTreeState() {
this.isClicked = !this.isClicked;
},
resetTreeState() {
this.isClicked = false;
},
},
mounted() {
const treeElement = document.getElementById('christmas-tree');
if (treeElement) {
treeElement.addEventListener('mouseleave', () => {
this.isClicked = false;
});
treeElement.addEventListener('click', () => {
this.isClicked = true;
});
}
},
};
</script>
Congratulations, the ChristmasTree.vue
is ready! 🎄✨ To actually display the component on the webpage, you need to add it to the App.vue:
import ChristmasTree from './components/ChristmasTree.vue';
…
<template>
<header>
<!-- Link to the WebDataRocks theme -->
<link rel="stylesheet" type="text/css" href="theme/teal/webdatarocks.min.css" />
<div class="main">
<div class="wrapper">
<div class="pivot-container">
<!-- Logo and Pivot Table -->
<div class="logo">📊 Vue 3 + WebDataRocks</div>
<Pivot v-bind:report="reportCsv" toolbar />
</div>
<!-- Christmas Tree Component -->
<ChristmasTree id="ch-tree" />
</div>
</div>
</header>
</template>
As you may have guessed, we will apply additional styles to the ChristmasTree component since there is its own id. The goal is to position the WebDataRocks Pivot Table and the ChristmasTree side by side. Check out these updated styles:
<style scoped>
#ch-tree {
margin-top: 13.5rem;
}
.pivot-container {
min-width: 1000px;
display: flex;
flex-direction: column;
}
:root {
--color-primary: #00796b;
--color-secondary: #004d40;
--color-text: #ffffff;
--color-background: #e0f2f1;
--color-border: #004d40;
}
header {
background: var(--color-primary);
color: var(--color-text);
padding: 1rem;
border-bottom: 3px solid var(--color-secondary);
}
.logo {
font-size: 1.5rem;
text-align: center;
font-weight: bold;
margin-bottom: 1rem;
}
nav a {
color: var(--color-text);
text-decoration: none;
padding: 0.5rem 1rem;
transition: all 0.3s ease;
}
nav a:hover {
background: var(--color-secondary);
border-radius: 4px;
}
nav a.router-link-exact-active {
font-weight: bold;
text-decoration: underline;
}
.wrapper {
display: flex;
flex-direction: column;
justify-content: space-between;
align-items: center;
height: fit-content;
gap: 10rem;
}
@media (min-width: 1024px) {
header {
display: flex;
align-items: center;
justify-content: space-between;
}
.wrapper {
display: flex;
flex-direction: row;
justify-content: space-between;
align-items: center;
}
nav {
margin-top: 0;
}
}
</style>
Let’s check what do we have so far:
To add the finishing touch with a border, create a separate style.css
file and add the following styles:
body {
border: 3em solid seashell;
border-top: 5em solid seashell;
border-bottom: 5em solid seashell;
height: fit-content;
}
Next, head over to your index.html
file and link this CSS file by adding the following line in the <head>
section:
<link rel="stylesheet" href="style.css" />
And there you have it! Save your code, run your project, and enjoy the result!
Final Words
And that’s it! You’ve built a festive data visualization dashboard with Vue 3 and WebDataRocks, complete with an interactive Christmas tree animation! 🎄✨
Our hope this project taught you some new skills and brought you a bit of holiday cheer. Feel free to customize the dashboard further with extra features to make it uniquely yours.
Oh and have you found any gift ideas? Share your thoughts in the comments!
Happy coding, and enjoy the holidays! 🎁
Flexmonster, with love.